Payment validation
Configure payment validation rules to send or accept payments based on your business requirements
Introduction
Payment validation is used to approve or reject payments before they are sent or when they are received. Common payment validations include checking:
- The status of an internal account before confirming or rejecting a payment received
- If a beneficiary is on a sanctions list before sending a payment
- If a direct debit mandate is not blocked when receiving a direct debit payment
- If a payment type and an amount are allowed for an internal account
Payment validation rules
Validations are managed via payment validation rules that group and orchestrate the execution of validations for a target payment flow (outgoing SCT Instant, incoming SDD, etc.)
A rule is defined by its:
- Criteria, defining the payments (payment orders or incoming payments) this rule will apply to. Supported criteria are connected accounts, payment types, and directions
- Validations which is the list of validations to be performed on these payments. Each check has a list of possible outcomes mapped to 3 different actions:
- Move to next validation
- Approve payment order (or confirm incoming payment)
- Cancel payment order (or reject incoming payment)
// Example of a payment validation rule for outgoing SCT Instant, checking whether the internal account is active and performing a PEP screening on the reciving account holder
{
"id": "7c149cf6-0306-4a45-b48c-b3562c39ab2b",
"object": "payment_validation_rule",
"name": "Internal account & PEP screening",
"scope": "payment_order",
"criteria": [
{
"attribute": "connected_account_id",
"extra": null,
"operator": "in",
"values": ["7c149cf6-0306-4a45-b48c-b3562c39ab2b"]
},
{
"attribute": "payment_direction",
"extra": null,
"operator": "equals",
"values": ["credit"]
},
{
"attribute": "payment_type","extra": null,
"operator": "equals",
"values": ["sepa_instant"]
}
],
"validations":[
[
{
"type": "is_internal_account_active",
"outcomes": [
{
"status": "successful",
"action": "next_validation"
},
{
"status": "failed",
"action": "cancel_payment"
}
]
}
],
[
{
"type": "pep_screening",
"outcomes": [
{
"status": "successful",
"action": "approve_payment"
},
{
"status": "failed",
"action": "cancel_payment"
}
]
}
]
]
}
Validations
Lifecycle
Validations have a status which reflects the progress made:
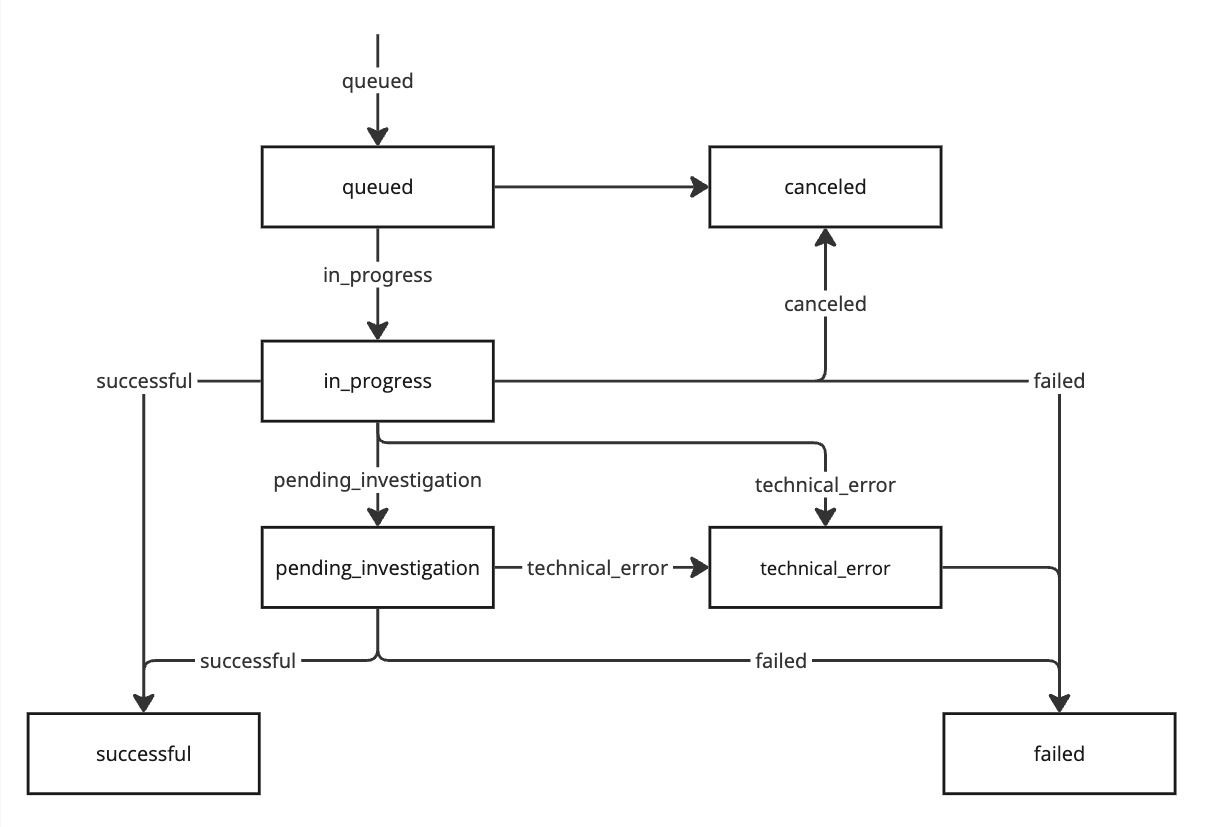
Types
Numeral currently supports two types of validations:
- Custom validations performed by your systems based on your internal logic and data providers
- Pre-built validations performed on Numeral objects and data, like internal accounts, direct debit mandates, external account reachability etc. which can be configured for your business needs
Custom validations
Numeral sends an event to your webhook when a validation in your system is required. The result of the validation can be shared using the dedicated API endpoint.
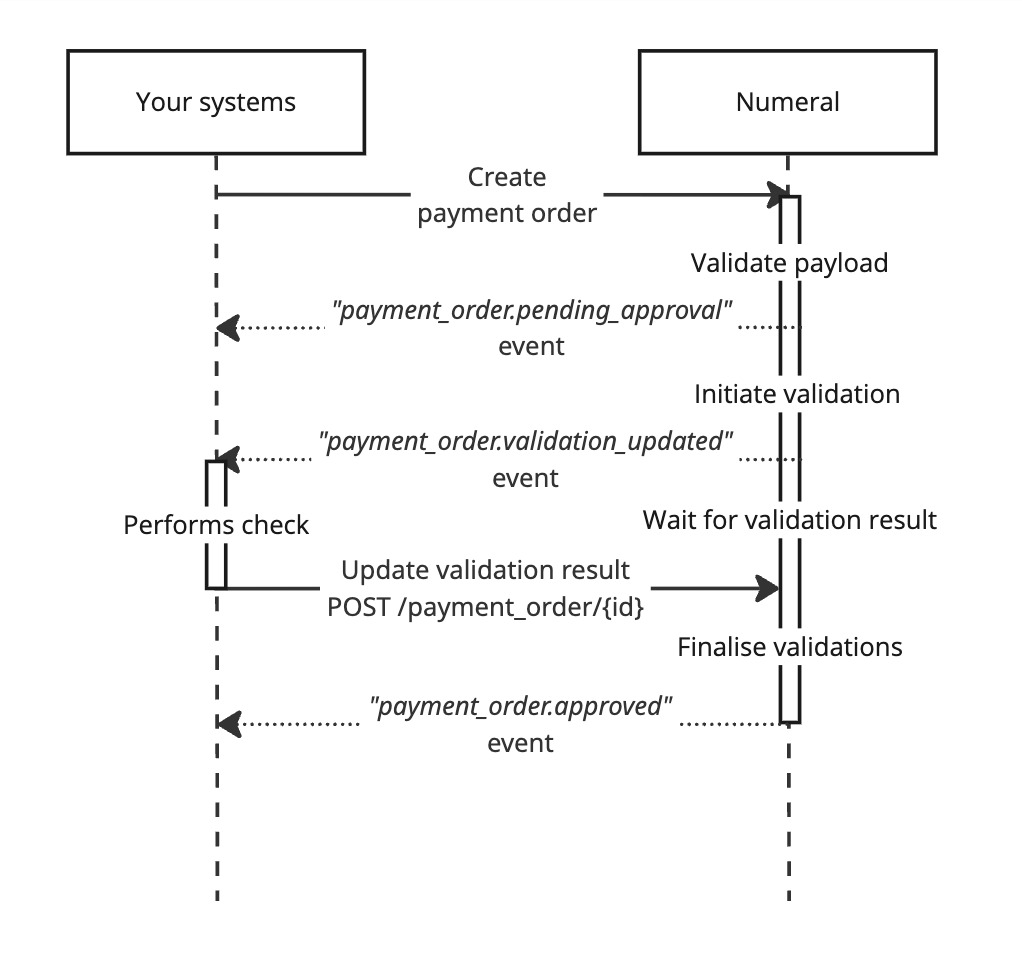
Pre-built validations
Numeral manages the validations based on your configuration.
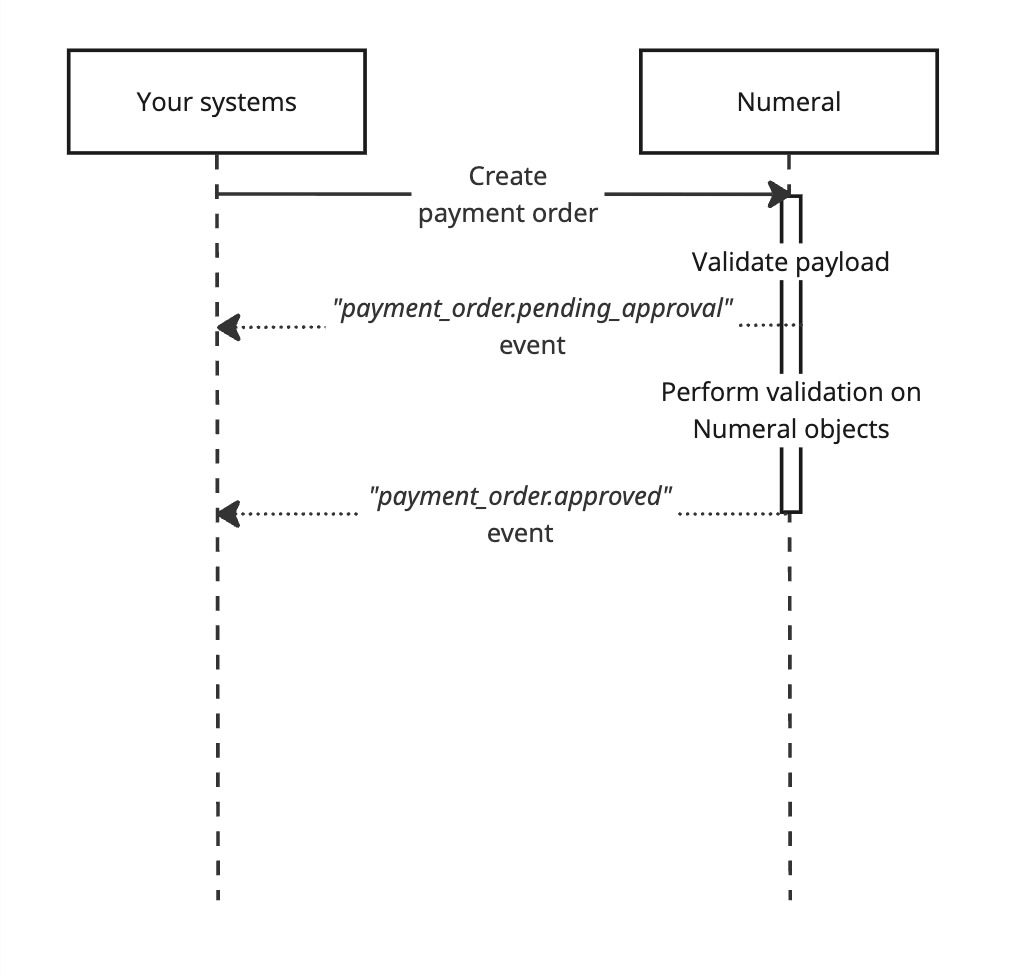
Payment validation flow
A payment order created or an incoming payment received include:
- A payment validation status
- The list of associated payment validation rules and, for each rule, a completion status and the list of associated validations with their status and result
Below is an example of a payment order with 2 payment validations:
// First validation of the internal account status is in progress
{
"id": "b38f5318-a389-4278-9cbe-d16116750987",
"object": "payment_order",
"status": "pending_apprvoal",
// Only a subset of the object is displayed to enhance clarity.
"payment_validation": {
"status": "in_progress",
"validation_results": [
{
"payment_validation_rule_id": "7c149cf6-0306-4a45-b48c-b3562c39ab2b",
"status": "in_progress",
"validations": [
[
{
"type": "internal_account_is_active",
"status": "in_progress",
"status_details": null,
"resource_id": "some ID",
"resource_url": "https://someurl.com/someid"
}
],
[
{
"type": "pep_screening",
"status": "queued",
"status_details": null,
"resource_id": null,
"resource_url": null
}
]
]
}
]
}
}
// First validation is successful. Second validation of PEP screening is in progress
{
"id": "b38f5318-a389-4278-9cbe-d16116750987",
"object": "payment_order",
"status": "pending_apprvoal",
// Only a subset of the object is displayed to enhance clarity.
"payment_validation": {
"status": "in_progress",
"validation_results": [
{
"payment_validation_rule_id": "7c149cf6-0306-4a45-b48c-b3562c39ab2b",
"status": "in_progress",
"validations": [
[
{
"type": "internal_account_is_active",
"status": "successful",
"status_details": null,
"resource_id": "some ID",
"resource_url": "https://someurl.com/someid"
}
],
[
{
"type": "pep_screening",
"status": "in_progress",
"status_details": null,
"resource_id": "some ID",
"resource_url": "https://someurl.com/someid"
}
]
]
}
]
}
}
// All validations are successful and payment is approved
{
"id": "b38f5318-a389-4278-9cbe-d16116750987",
"object": "payment_order",
"status": "approved",
// Only a subset of the object is displayed to enhance clarity.
"payment_validation": {
"status": "successful",
"validation_results": [
{
"payment_validation_rule_id": "7c149cf6-0306-4a45-b48c-b3562c39ab2b",
"status": "successful",
"validations": [
[
{
"type": "internal_account_is_active",
"status": "successful",
"status_details": null,
"resource_id": "some ID",
"resource_url": "https://someurl.com/someid"
}
],
[
{
"type": "pep_screening",
"status": "successful",
"status_details": null,
"resource_id": "some ID",
"resource_url": "https://someurl.com/someid"
}
]
]
}
]
}
}
Updated 27 days ago